11.1.6. Binary MPC Example¶
Let us consider a simple MPC example where the system has inputs that can take only two values, \(u_{min}\) or \(u_{max}\). The original problem (shown on the left) can be reformulated into the problem on the right, which corresponds to a standard form for which FORCESPRO can generate a solver. The details of the reformulation are given at the end of this example.
Simple MPC problem with discrete inputs:
Equivalent problem with binary inputs
The problem on the right can now be easily formulated in FORCESPRO. Note that the problem description is very similar to that of the simple MPC example, with the only modification that certain variables are marked to be binary. Download and run a complete simulation script to see the output.
nx = 2; nu = 2;
% assume variable ordering zi = [delta_{i-1}; x{i}] for i=1...N
stages = MultistageProblem(N);
for i = 1:N
% dimension
stages(i).dims.n = nx+nu; % number of stage variables
stages(i).dims.r = nx; % number of equality constraints
stages(i).dims.l = nx; % number of lower bounds
stages(i).dims.u = nx; % number of upper bounds
stages(i).bidx = 1:nu; % index of binary variables
% cost
if( i == N )
stages(i).cost.H = blkdiag(Rtilde,P);
else
stages(i).cost.H = blkdiag(Rtilde,Q);
end
stages(i).cost.f = [ftilde; zeros(nx,1)];
% lower bounds
stages(i).ineq.b.lbidx = (nu+1):(nu+nx); % lower bound on states
stages(i).ineq.b.lb = xmin; % upper bound values
% upper bounds
stages(i).ineq.b.ubidx = (nu+1):(nu+nx); % upper bound for this stage variable
stages(i).ineq.b.ub = umax; % upper bound for this stage variable
% equality constraints
if( i < N )
stages(i).eq.C = [zeros(nx,nu), A];
end
if( i>1 )
stages(i).eq.c = -Bconst;
end
stages(i).eq.D = [Btilde, -eye(nx)];
end
% RHS of first eq. constr. is a parameter: z1=-A*x0
params(1) = newParam('minusA_times_x0',1,'eq.c');
You can download the MATLAB code of this example to try it out for yourself by
clicking here
.
You can download the Python code of this example to try it out for yourself by
clicking here
.
11.1.6.1. Simulation result¶
When running the example, you should see the following closed-loop behavior:
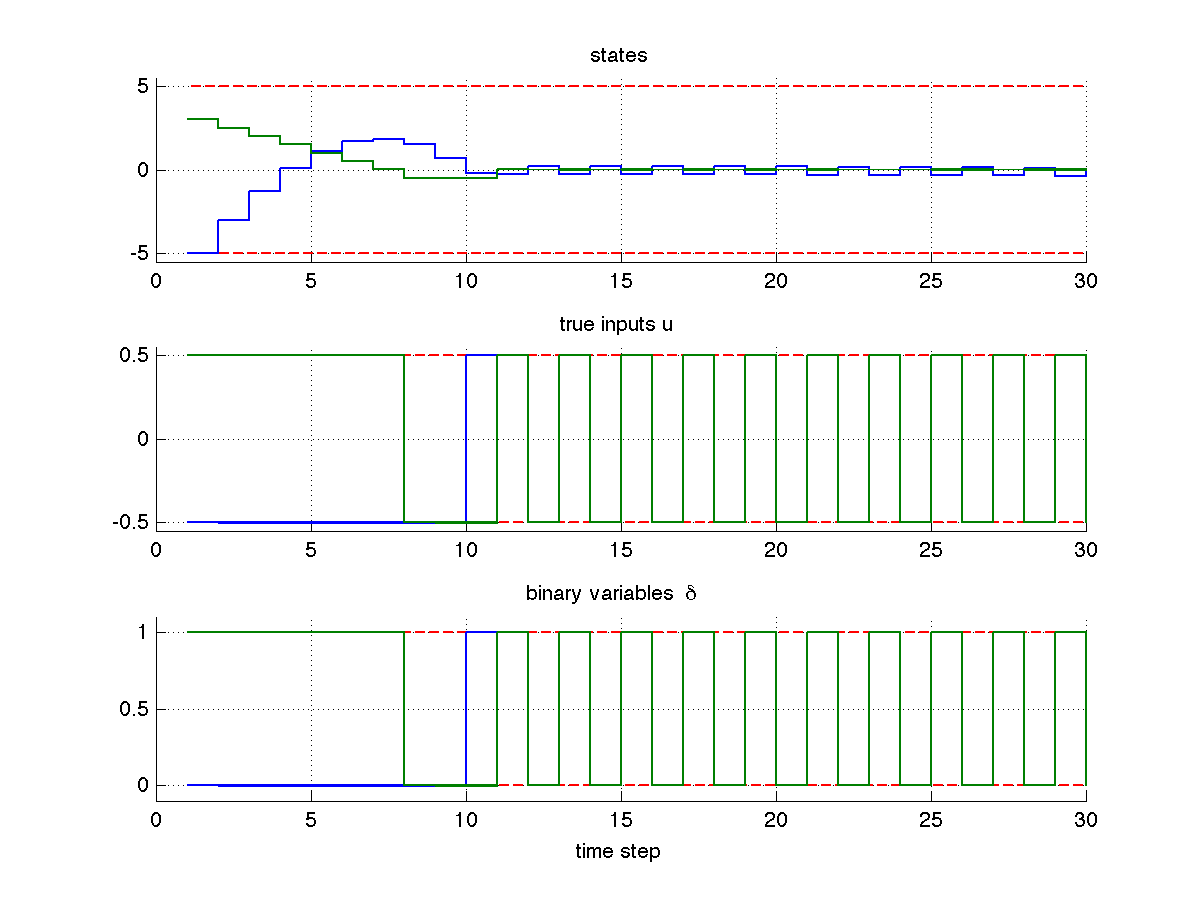
11.1.6.2. Details on problem reformulation¶
The reformulation is done as follows: we introduce a variable \(delta\) such that
This can be formulated by the equality constraint
where \(\text{diag}\) denotes a diagonal matrix. To keep the number of variables at a minimum, we will directly insert this equation into the dynamics:
where \(\tilde{B}:=B \text{diag}(u_{max}-u_{min})\) and \(b:=B u_{min}\).
Similarly for the cost function,
where